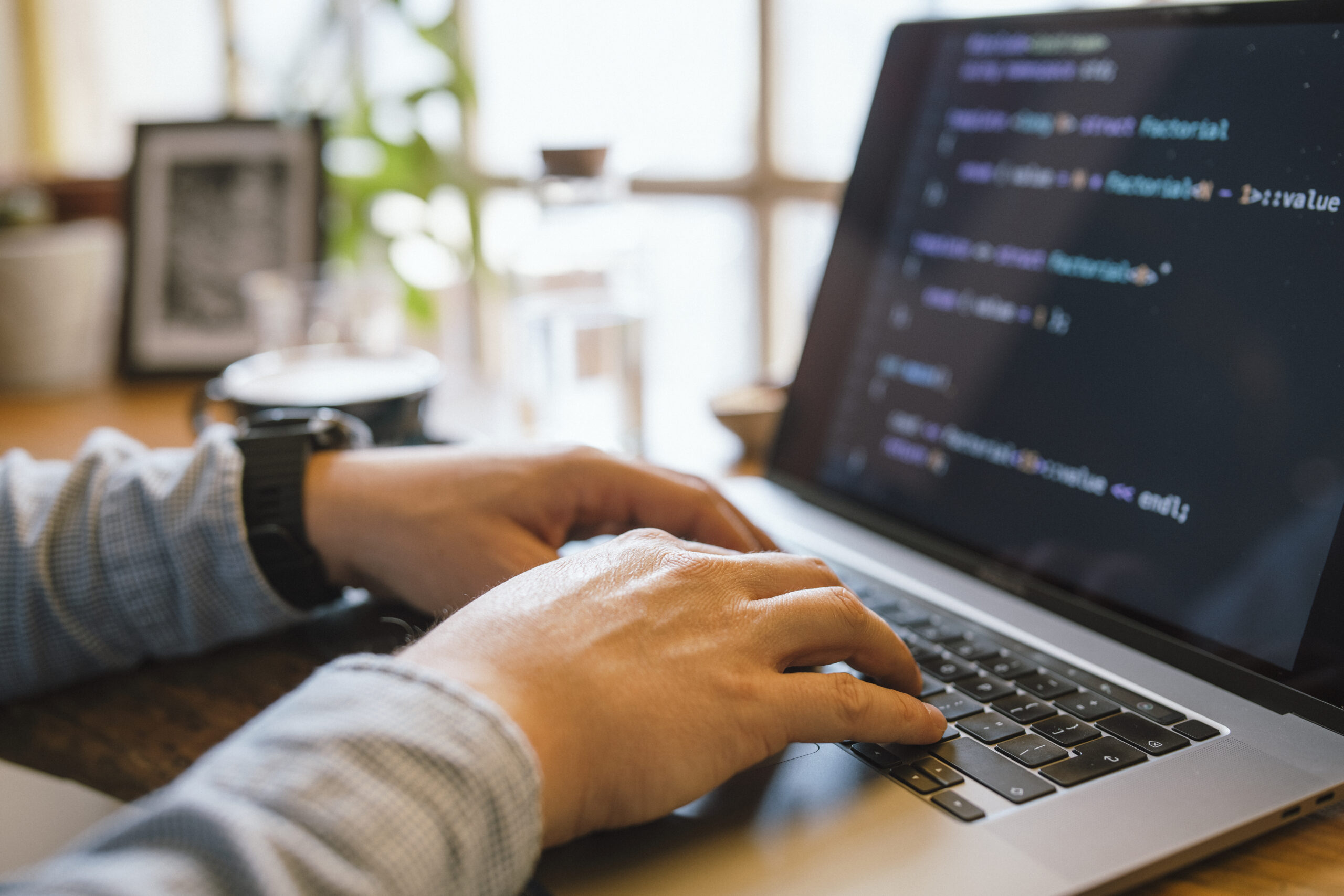
Debugging is Just about the most essential — nevertheless generally missed — abilities within a developer’s toolkit. It's actually not pretty much correcting damaged code; it’s about being familiar with how and why things go Incorrect, and Understanding to Feel methodically to resolve troubles proficiently. No matter if you are a novice or maybe a seasoned developer, sharpening your debugging techniques can help save hrs of stress and substantially increase your productivity. Here i will discuss a number of techniques to aid builders stage up their debugging recreation by me, Gustavo Woltmann.
Grasp Your Equipment
One of many fastest strategies builders can elevate their debugging capabilities is by mastering the resources they use daily. Although creating code is one Element of progress, figuring out the way to interact with it effectively all through execution is Similarly essential. Modern progress environments arrive equipped with highly effective debugging capabilities — but numerous builders only scratch the surface area of what these applications can perform.
Consider, such as, an Integrated Development Environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These tools assist you to set breakpoints, inspect the worth of variables at runtime, phase through code line by line, and in many cases modify code around the fly. When made use of appropriately, they let you observe accurately how your code behaves for the duration of execution, which is priceless for monitoring down elusive bugs.
Browser developer resources, which include Chrome DevTools, are indispensable for front-conclusion developers. They enable you to inspect the DOM, monitor network requests, perspective actual-time performance metrics, and debug JavaScript while in the browser. Mastering the console, resources, and network tabs can switch disheartening UI concerns into workable responsibilities.
For backend or program-stage builders, resources like GDB (GNU Debugger), Valgrind, or LLDB provide deep Management over working procedures and memory administration. Learning these equipment could possibly have a steeper learning curve but pays off when debugging efficiency difficulties, memory leaks, or segmentation faults.
Further than your IDE or debugger, turn out to be cozy with Model Command systems like Git to grasp code heritage, obtain the exact moment bugs had been launched, and isolate problematic alterations.
In the long run, mastering your applications means going past default settings and shortcuts — it’s about building an intimate understanding of your growth natural environment to make sure that when issues arise, you’re not lost in the dark. The better you realize your resources, the more time you are able to invest solving the actual difficulty as an alternative to fumbling by way of the method.
Reproduce the situation
Among the most important — and sometimes forgotten — techniques in powerful debugging is reproducing the challenge. Just before jumping into the code or earning guesses, builders have to have to produce a regular setting or situation where the bug reliably seems. Devoid of reproducibility, repairing a bug becomes a activity of chance, normally leading to wasted time and fragile code improvements.
Step one in reproducing a problem is accumulating as much context as possible. Check with inquiries like: What actions brought about the issue? Which ecosystem was it in — growth, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The more element you've got, the easier it will become to isolate the exact disorders beneath which the bug takes place.
As soon as you’ve gathered ample information, endeavor to recreate the issue in your neighborhood ecosystem. This might necessarily mean inputting precisely the same data, simulating related person interactions, or mimicking program states. If The difficulty appears intermittently, take into account writing automated assessments that replicate the edge circumstances or point out transitions involved. These exams don't just assist expose the situation but also avoid regressions Down the road.
Occasionally, The problem can be atmosphere-certain — it'd happen only on specified functioning systems, browsers, or below distinct configurations. Applying tools like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating such bugs.
Reproducing the trouble isn’t merely a action — it’s a attitude. It involves tolerance, observation, and a methodical method. But after you can persistently recreate the bug, you happen to be by now midway to correcting it. Which has a reproducible state of affairs, you can use your debugging tools more successfully, check prospective fixes safely and securely, and converse far more Plainly using your crew or end users. It turns an abstract complaint into a concrete challenge — Which’s where by builders prosper.
Read through and Recognize the Error Messages
Error messages are often the most valuable clues a developer has when a little something goes Completely wrong. Rather then looking at them as annoying interruptions, developers ought to learn to take care of mistake messages as direct communications in the system. They normally show you just what exactly occurred, exactly where it transpired, and from time to time even why it occurred — if you know how to interpret them.
Get started by looking at the message carefully As well as in total. Many builders, particularly when under time force, glance at the first line and promptly commence making assumptions. But further within the mistake stack or logs might lie the legitimate root lead to. Don’t just copy and paste mistake messages into search engines like yahoo — read and fully grasp them 1st.
Break the mistake down into components. Can it be a syntax error, a runtime exception, or possibly a logic error? Does it issue to a particular file and line selection? What module or operate brought on it? These queries can guideline your investigation and level you towards the responsible code.
It’s also valuable to understand the terminology with the programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java generally adhere to predictable designs, and Studying to acknowledge these can drastically accelerate your debugging system.
Some mistakes are obscure or generic, As well as in These situations, it’s very important to examine the context through which the mistake occurred. Examine linked log entries, enter values, and recent adjustments from the codebase.
Don’t overlook compiler or linter warnings both. These typically precede larger sized problems and provide hints about likely bugs.
Finally, mistake messages aren't your enemies—they’re your guides. Understanding to interpret them effectively turns chaos into clarity, encouraging you pinpoint problems more rapidly, lower debugging time, and turn into a extra efficient and confident developer.
Use Logging Wisely
Logging is Just about the most strong instruments in a very developer’s debugging toolkit. When made use of effectively, it provides actual-time insights into how an application behaves, aiding you fully grasp what’s going on under the hood without needing to pause execution or step through the code line by line.
A good logging strategy starts with knowing what to log and at what amount. Popular logging concentrations contain DEBUG, Information, Alert, Mistake, and Deadly. Use DEBUG for in depth diagnostic info throughout development, INFO for typical gatherings (like profitable start off-ups), WARN for potential challenges that don’t crack the appliance, ERROR for precise challenges, and Deadly once the system can’t go on.
Prevent flooding your logs with extreme or irrelevant information. Too much logging can obscure vital messages and slow down your system. Deal with essential activities, point out alterations, input/output values, and important determination points as part of your code.
Format your log messages Evidently and persistently. Consist of context, which include timestamps, request IDs, and performance names, so it’s easier to trace challenges in distributed units or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even easier to parse and filter logs programmatically.
For the duration of debugging, logs Allow you to observe how variables evolve, what situations are achieved, and what branches of logic are executed—all devoid of halting the program. They’re In particular beneficial in generation environments exactly where stepping by code isn’t feasible.
On top of that, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about stability and clarity. That has a nicely-considered-out logging approach, you'll be able to lessen the time it takes to spot difficulties, gain deeper visibility into your apps, and Increase the General maintainability and dependability of your respective code.
Think Like a Detective
Debugging is not simply a complex task—it's a kind of investigation. To proficiently identify and repair bugs, builders should tactic the procedure like a detective fixing a mystery. This attitude can help stop working elaborate problems into manageable elements and comply with clues logically to uncover the foundation cause.
Begin by gathering evidence. Consider the indicators of the situation: mistake messages, incorrect output, or efficiency troubles. The same as a detective surveys against the law scene, accumulate just as much suitable information and facts as you could without jumping to conclusions. Use logs, test cases, and user experiences to piece alongside one another a transparent photo of what’s occurring.
Following, kind hypotheses. Check with on your own: What may very well be resulting in this habits? Have any alterations just lately been created for the codebase? Has this problem occurred right before less than very similar conditions? The aim would be to slender down alternatives and establish likely culprits.
Then, test your theories systematically. Try to recreate the condition in a very controlled environment. For those who suspect a certain perform or ingredient, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, question your code concerns and Enable the outcome lead you nearer to the truth.
Pay back near interest to compact information. Bugs usually hide from the minimum envisioned spots—like a lacking semicolon, an off-by-1 mistake, or perhaps a race affliction. Be comprehensive and affected individual, resisting the urge to patch the issue without the need of completely being familiar with it. Short term fixes may conceal the actual difficulty, only for it to resurface afterwards.
Lastly, hold notes on what you experimented with and acquired. Just as detectives log their investigations, documenting your debugging course of action can conserve time for long run difficulties and help Other folks have an understanding of your reasoning.
By pondering similar to a detective, builders can sharpen their analytical expertise, tactic problems methodically, and grow to be simpler at uncovering concealed issues in advanced systems.
Compose Assessments
Crafting tests is one of the best solutions to improve your debugging abilities and Total progress performance. Checks not only aid catch bugs early but in addition function a security Web that offers you confidence when producing alterations to the codebase. A very well-analyzed software is much easier to debug mainly because it allows you to pinpoint precisely in which and when an issue happens.
Begin with unit exams, which target particular person capabilities or modules. These compact, isolated checks can quickly reveal whether or not a specific piece of logic is working as expected. When a test fails, you straight away know where by to glimpse, noticeably cutting down enough time put in debugging. Unit exams are Particularly beneficial for catching regression bugs—problems that reappear following Beforehand staying fastened.
Following, integrate integration checks and conclusion-to-conclude exams into your workflow. These help make sure several areas of your application do the job collectively smoothly. They’re particularly handy for catching bugs that arise in complicated units with a number of components or products and services interacting. If anything breaks, your tests can show you here which Portion of the pipeline unsuccessful and beneath what conditions.
Producing exams also forces you to definitely Feel critically regarding your code. To test a characteristic thoroughly, you require to know its inputs, envisioned outputs, and edge circumstances. This volume of knowing The natural way qualified prospects to raised code construction and less bugs.
When debugging an issue, composing a failing exam that reproduces the bug could be a robust first step. After the exam fails regularly, you may concentrate on repairing the bug and check out your check move when The difficulty is resolved. This strategy makes certain that the same bug doesn’t return Later on.
Briefly, writing exams turns debugging from a discouraging guessing game into a structured and predictable approach—serving to you capture much more bugs, more quickly plus much more reliably.
Choose Breaks
When debugging a tricky concern, it’s effortless to be immersed in the condition—staring at your screen for hours, making an attempt Resolution immediately after solution. But Just about the most underrated debugging equipment is actually stepping absent. Getting breaks will help you reset your head, lower irritation, and infrequently see The difficulty from the new standpoint.
If you're much too near the code for much too extensive, cognitive exhaustion sets in. You may perhaps get started overlooking noticeable faults or misreading code that you choose to wrote just several hours before. With this point out, your Mind turns into significantly less effective at difficulty-solving. A brief wander, a espresso split, or perhaps switching to a different task for ten–quarter-hour can refresh your target. Several developers report finding the foundation of a challenge once they've taken time for you to disconnect, letting their subconscious get the job done while in the track record.
Breaks also assist prevent burnout, Primarily through more time debugging sessions. Sitting down in front of a display, mentally trapped, is not simply unproductive but additionally draining. Stepping away means that you can return with renewed Strength along with a clearer mentality. You would possibly abruptly notice a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you right before.
In case you’re stuck, a fantastic rule of thumb should be to set a timer—debug actively for forty five–60 minutes, then have a 5–ten minute split. Use that point to move all-around, stretch, or do a little something unrelated to code. It may well truly feel counterintuitive, especially beneath limited deadlines, nonetheless it actually contributes to faster and simpler debugging Ultimately.
In a nutshell, having breaks isn't an indication of weak spot—it’s a smart tactic. It gives your brain Place to breathe, improves your viewpoint, and can help you avoid the tunnel vision That always blocks your development. Debugging is really a psychological puzzle, and relaxation is part of solving it.
Study From Each Bug
Every single bug you encounter is much more than simply A short lived setback—It is a chance to improve as a developer. Regardless of whether it’s a syntax mistake, a logic flaw, or maybe a deep architectural issue, each one can educate you a thing important in the event you make time to mirror and assess what went wrong.
Begin by asking oneself a number of critical thoughts once the bug is resolved: What brought on it? Why did it go unnoticed? Could it have already been caught previously with greater techniques like device screening, code testimonials, or logging? The solutions generally expose blind places with your workflow or knowledge and make it easier to Establish much better coding patterns going ahead.
Documenting bugs can even be an outstanding practice. Hold a developer journal or keep a log where you Be aware down bugs you’ve encountered, how you solved them, and what you learned. Over time, you’ll begin to see styles—recurring troubles or frequent blunders—that you could proactively steer clear of.
In team environments, sharing Anything you've figured out from the bug along with your peers is usually In particular strong. Regardless of whether it’s through a Slack information, a brief compose-up, or A fast know-how-sharing session, supporting Other people steer clear of the similar concern boosts team performance and cultivates a more powerful learning lifestyle.
A lot more importantly, viewing bugs as classes shifts your frame of mind from disappointment to curiosity. Instead of dreading bugs, you’ll start out appreciating them as important aspects of your growth journey. In the end, a lot of the greatest builders will not be those who compose perfect code, but individuals who continuously study from their errors.
In the long run, Every bug you deal with adds a whole new layer to your ability established. So subsequent time you squash a bug, have a instant to reflect—you’ll appear absent a smarter, much more able developer because of it.
Conclusion
Increasing your debugging skills normally takes time, observe, and patience — even so the payoff is large. It tends to make you a far more economical, confident, and capable developer. The subsequent time you might be knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be much better at Whatever you do.